Help compiling preact
Here's my current setup:
I'm compiling it using esbuild as follows:
This ultimately gets compiled down to the file that I've attached. Unfortunately, when I actually try to run the code I get the error message I've attached. Any ideas?
10 Replies
test html page for fun:
cc @marvinh. if you could give some guidance when you get the chance
I see
write: false
-- is that script not writing files, and you're still running an old version?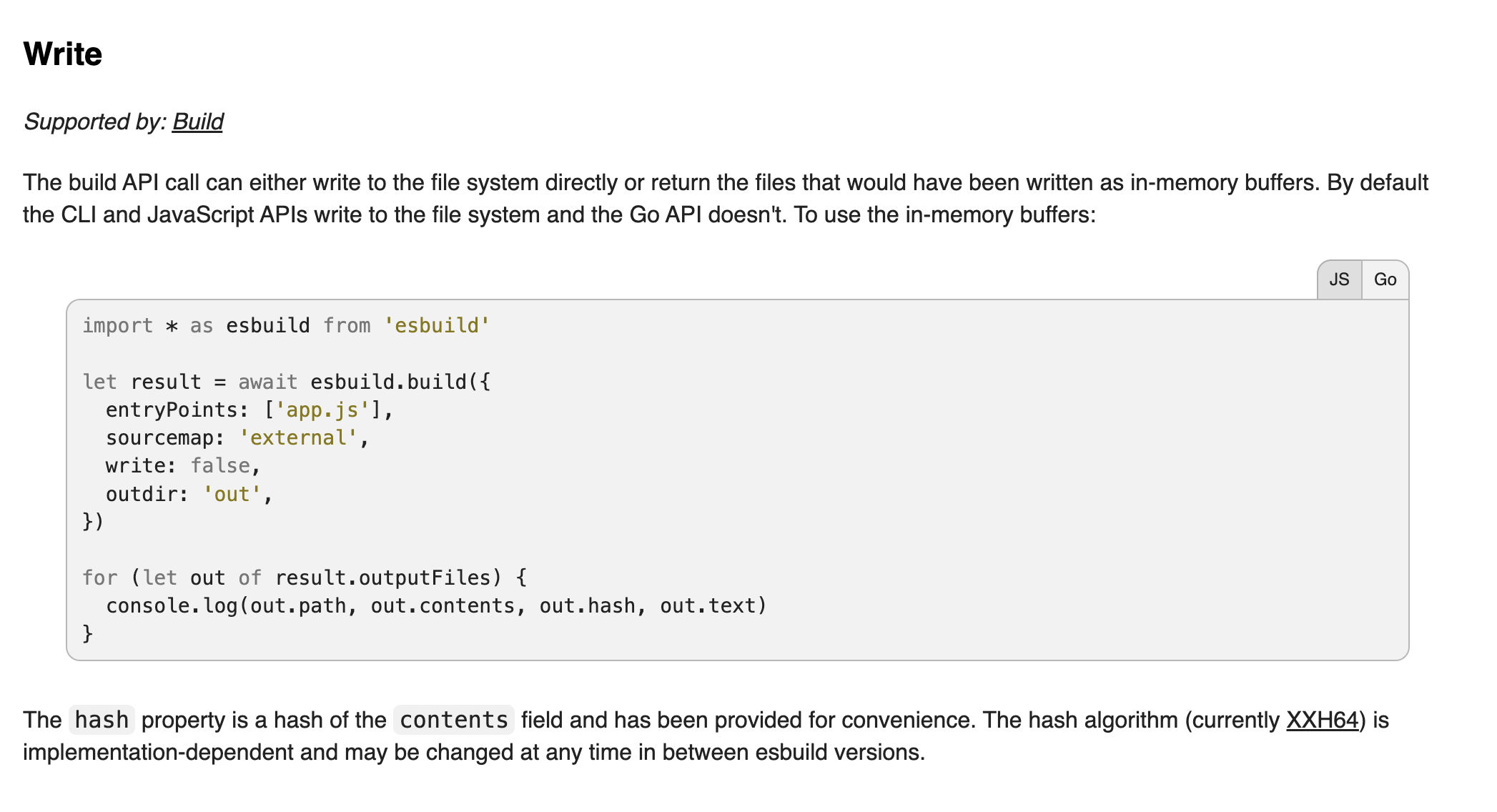
I write to the filesystem outside of the included reproduction
Weird. I use esbuild (indirectly) to compile and embed preact code and haven’t run into this problem.
would love to see your setup
https://github.com/diskuto/diskuto-web/blob/main/embedder.ts
It’s a bit indirect. It’s using deno-embedder to embed files. But internally that uses esbuild.
I think you need to load your script with
type="module"
instead of type="text/javascript"
because the output format is in ESM. I was able to run the example above with:
You also need to run some local server to avoid security error in browser
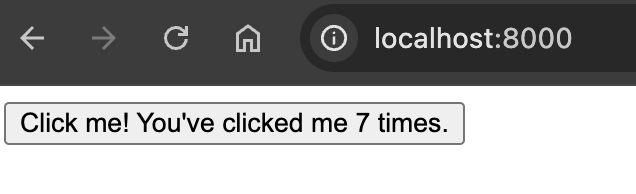
🙏
Thanks! I should have tried that earlier