Questions about files and what happens when other programs writes to them
I am a noob at programming. There is this video game I play named Team Fortress 2 and it has this option called condebug which makes it create a file with all of the contents of the in-game console, this file is updated in realtime. I'm going to use this in deno to detect in-game button presses and team-changes to control VLC Media Player. I want to make a script with Deno that reads this file out in realtime and decodes it to perform actions when it sees certain groups of text, I am wondering what happens if Team Fortress 2 writes to the file at the same exact time that Deno tries to read it? Does my computer explode? Does Deno get some sort of incomplete output that would make my program mis-interpret what it read? Or does nothing bad happen at all? Also I'm wondering what Deno.close does, I want the file to be endlessly read until the script is closed, so do I repeatedly open and close the file or just keep it open until the script closes (is it even possible to close the file when the script closes?)?
41 Replies
I am wondering what happens if Team Fortress 2 writes to the file at the same exact time that Deno tries to read itI think it depends on how the game edits the file. The file might be locked or not. Also the file might not exist if the game replaces it instead of editing Does the game only append content to the file or does it edit the lines that already were in the file?
it only appends content unless you have the option "conclearlog" which clears the file once as soon as the game starts up
Whats your operating system?
Windows 11, but keeping compatibility with Windows 10 and Linux would be nice I think probably
On Linux and Mac you could use the command
tail -f
to keep reading the file. On Windows I don't think it worksOh but I'm asking about the functions like fsfile.close and fsfile.readable
this thing is what I'm supposed to use right? https://docs.deno.com/api/deno/~/Deno.FsFile
Deno
Deno.FsFile - Deno - Deno Docs
In-depth documentation, guides, and reference materials for building secure, high-performance JavaScript and TypeScript applications with Deno
When does a file lock happen and what would it mean for my script?
I think your program will exit when it finishes reading the file. It wont remain open. Thats why
tail -f
would be handyI just want to use readable or a loop to keep reading it endlessly until I close Team Fortress 2, or VLC, or the command prompt. I don't intend on it stopping after the file is read once
Yep
So if you cant
tail -f
, you'll probably have to keep reopening the fileWhy can't I leave the file open and use the readable?
Maybe you can, but you'll need some sort of timer I think
If you just leave it in an infinite loop, I'm afraid it will consume a lot of CPU
I dont think it would consume a lot of cpu I mean the example they use seems to use an infinite loop of some sorts
Thats not infinite. It ends when the file ends
oh
You don't want your loop to end. You want to wait for more edits. Thats the difference
This one is infinite: https://docs.deno.com/api/deno/~/Deno.watchFs
You might need to use it to detect when the file changes
Deno
Deno.watchFs - Deno - Deno Docs
In-depth documentation, guides, and reference materials for building secure, high-performance JavaScript and TypeScript applications with Deno
their example doesn't really seem to work for me anyways >.>
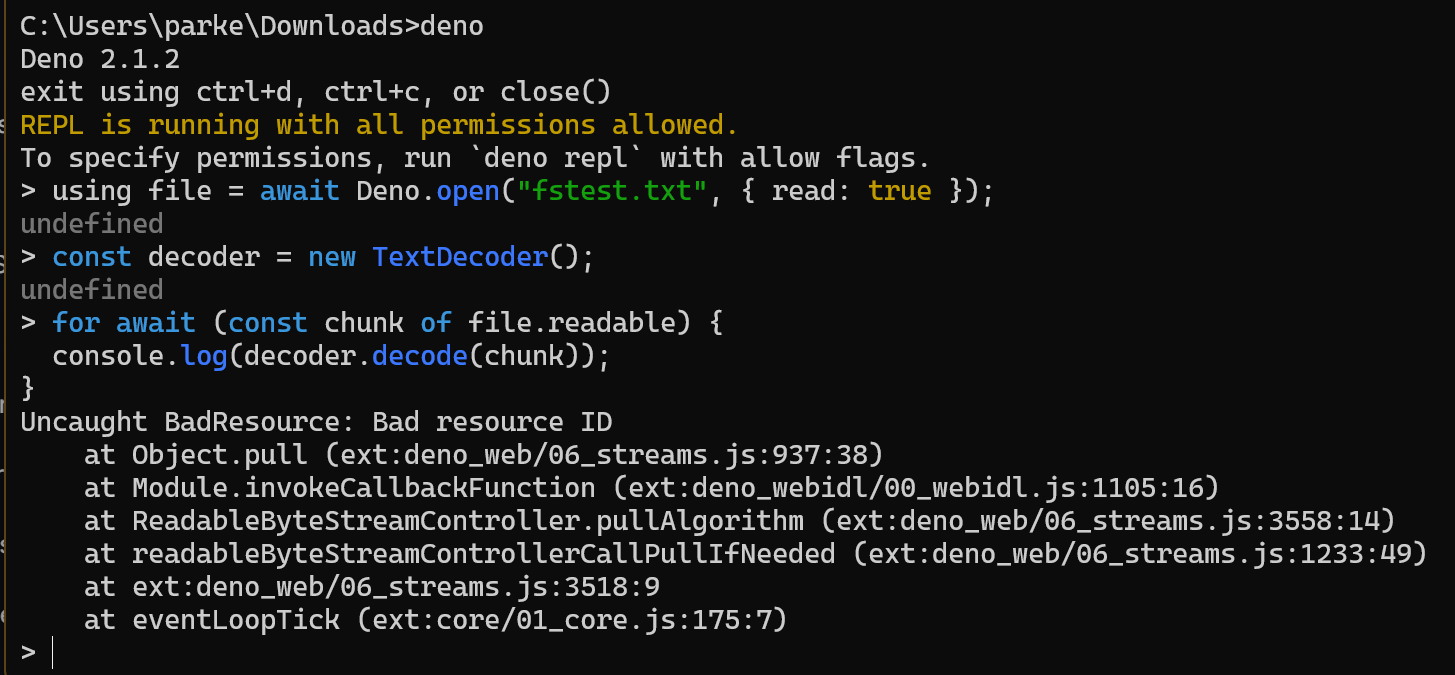
try using
let file
instead of using file
Just make two programs to test its functionality.
One program to slowly make writes to it via a stream and another one to read from it via a stream.
If the read program exits while the write program is still going then it doesn't work
im still not sure what the close does and when I should use it
If you weren't using a readable stream and using the other read and write functions on it, then you'd call close to indicate that the file can be released. Otherwise it just hangs around in memory.
The close function is to indicate you're finished interacting with the file.
When working with readable and writable streams on the file, they automatically call this function when they are closed themselves.
An alternative is to use the tail command on Linux and macOS and on windows use
Get-Content -Path "path\to\your\file.txt" -Wait
(according to ChatGPT). Both via the Deno.Command. You can stream their outputs.will anything bad happen if my script crashes before I close the file or the stream?
No. When programs crash the OS is designed to clean up any resources they were using.
That doesn't always apply to child processes though. Depends on the child.
Is this proper way to read out all the new content (readNewLines is in a timer)
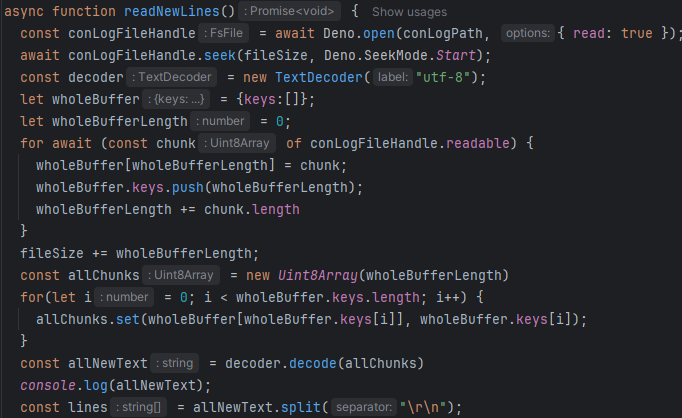
Why am I getting BadResource after seeking a FsFile after reading it with readable?
it wont let me use close on it either
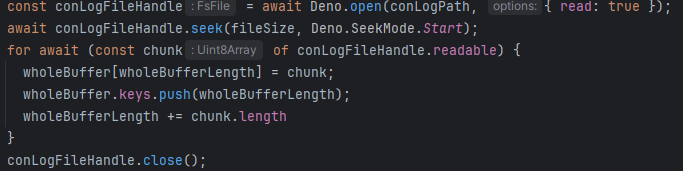

File is already closed
Why is it closed even if I don't call close()?
Because the readable stream property called closed when it finished.
even when I use preventCancel?
I've only seen that for a writable stream
MDN Web Docs
ReadableStream - Web APIs | MDN
The ReadableStream interface of the Streams API represents a readable stream of byte data. The Fetch API offers a concrete instance of a ReadableStream through the body property of a Response object.
Cancel and close are different.
Prevent cancel is stopping the readable from being closed early if the for loop breaks out early.
It will still close itself when its exhausted
oh hmm
how do I prevent this
Why do you want to prevent it? You literally close it afterwards
the close was just the example I was wondering if I could leave the file open so I could continue reading it later when it's modified instead of opening and closing it again
You could try that with a reader.
readable.getReader()
But idk if it would work
yeah i dont think that works