KV multimap / unordered keys search
Hello,
I want to do an unordered keys search on deno kv
FoundationDB talk about multimap (https://apple.github.io/foundationdb/multimaps.html ). I don't find it for deno kv.
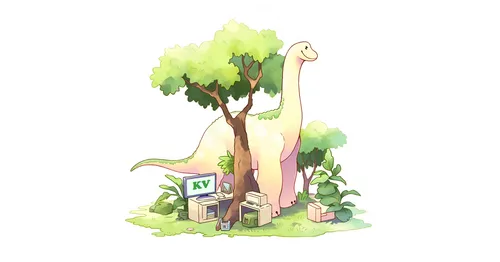
4 Replies
what do you mean unordered? do you mean arbitrarily ordered?
in my example, I want to get all keys including
["users", "basket"]
or ["basket", "users"]
, ie I don't care for orders.
kv only authorises strict prefix, here only ["users","alex"]
works
In a real usecase, I want to filter on some criteria (country, city, sport...)the article you shared is unrelated to the behavior you want
this is something you can achieve by creating your own secondary indices
the code example shows that you can't do it with prefix, only if your criterias meet exactly your key