Log output from Deno.ChildProcess while its running
I want to log all output immediately when its logged in the child process. how would i do that?
19 Replies
@Kay here this should help you
if you have more questions please let me know
if you don't need spawn()
then its simpler:
as with that the stdout is piped by default
i do need to spawn it but i dont want to wait for the output. is there a way to get live output from the process?
yes you can use WHATWG Stream for that
whats that and how do i implement it?
you can get the stream of stdout (which itself is a stream)
and pipe and modify it.
we get raw binary as stream but we want that a string stream so we can print it out or do other stuff with it
the deno std also has stream helpers like a csv stream parser or json stream parser when you are parsing multiple lines
i did a quick search and stackoverflow said i also could use
inherit
for the stdout and stderr. whats the difference?inherit means it uses the same stdout as deno
so if you process prints out Hello World deno would print our Hello World
yhea the process im running is deno so that should be fine right?
but because its not pipe though "javascript" you itself can't read it
ohh
yes, but btw if you subprocess is deno again maybe look into workers
typo*
https://docs.deno.com/runtime/manual/runtime/workers there are great docs about it
Workers | Deno Docs
Deno supports
yhea im just using Deno.Command to easely generate the command. i want to limit it to only the apis it needs and nothing else and to create a huge list in the deno.json file is a bit cluttered so i wanted to just generate it and store it in a way that can be read and modified better
oh so maybe you want to look into deno tasks?
couse im pretty sure there is no other way to specify allows and denies other than in the tasks
you just write deno task stat and it runns the command in the screenshot
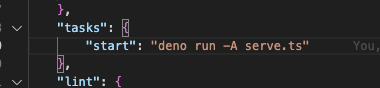
i did. its just that a huge list of websites in the command is a pain to read and modify
ahh okay i see
so id have smth like
--allow-net=discord.com,gateway.discord.com,deno.land,0.0.0.0,someotherthing.net
and so on