What am I missing when conditionally styling SVGs
So I have a SVG of an arrow that only has a stroke color. I want to change this stroke color based on a bool value passed to the component, if true color = black else white.
Desired output (attached photo):
Renders a svg that doesn't show:
What other info should I include here to better help others help me understand deno/fresh rendering better?
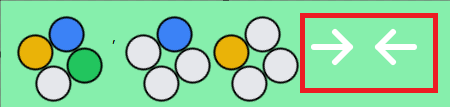
3 Replies
I wasn't able to find the answer to your question in the official Deno documentation. However, based on the code snippet you've provided, I can offer some general advice.
In the second code snippet where you're trying to conditionally apply the stroke color, you're using template literals to set the class name. This should work as expected, assuming that the
hold
variable is a boolean and is being passed correctly to the component. However, if the SVG is not rendering as expected, here are a couple of things you might want to check:
1. Ensure that the CSS classes w-12
, h-12
, stroke-white
, and stroke-black
are defined in your stylesheet and are being applied correctly. If these classes are not defined, the SVG might not be visible due to having no width, height, or stroke color.
2. Check if the conditional logic for the class is working as intended. You can temporarily replace the template literal with a static class name to see if the SVG renders correctly.
3. Make sure that the DirectionalCommandVariants
type (if it's TypeScript) correctly defines the hold
property as a boolean.
4. Verify that there are no console errors in the browser's developer tools that could indicate a problem with the rendering.
Please note that this advice is not based on the Deno documentation, and I would advise you to double-check the accuracy of the advice given. If the issue persists, you may want to seek help from the community or look for examples of similar implementations for further guidance.use full classes
It's not really a fresh issue
that's how tailwind works
Will try this and update with results, thanks for the response.
Thanks that was the answer! Worked exactly how I expected it too.