Get image from automated download
I have this page which displays a render using threejs and renders it into a png and downloads it. i need that image to use it in a canvas from deno-canvas but when i download it using this package it returns this:
code where i download it:|
21 Replies
Looking at the docs of the
download
library the file
parameter is there to set the filename only. You're passing a full absolute path into that. Under the hood the library seem to only append the file
parameter to their own internal path leading to the broken file path you're seeing in the error message.
You might have better luck just using builtin APIs to download a file:
this still gives an error
new file:
it does look like progress tho
@Kay ah apologies, the
file.close()
should be removed
Deleted it from my code snippet. Not sure why I added that, my brain blanked probablythat worked. only the image is not valid. the
stone-valid.png
is downloaded by me just going to the url and the stone-invalid.png
is downloaded by the code. the file sizes do not match and the invalid one does not load in any image editor/viewer
marvinh’s code works for me. Are you sure the file being served is the same?
its not exactly being served. its generated. first threejs generates a cube and loads a texture from a material from the url parameters. then it generates an image and downloads it
sounds like something is wrong with how the image is generated
could be but if i just go to the page myself it works fine
this is the renderer
https://gist.github.com/phant0m2290/bf07e2cf7c020879e3dfc6b8e3a089e4
It seems like you’re actually downloading the page’s source instead of the image you want.
I’m not sure you can easily/seamlessly just have Deno save the file from the browser JavaScript code.
ahh ye when i opened the file i do indeed see the html
But note that you can just run that code on Deno itself if you use the right tools, instead of having it run on a browser.
I guess Three.js might not be possible, actually. 🤔
yhea only i coudnt find a local kind of version of threejs or something like it
If you just want an isometric image, you could create it without Three.js.
how do you mean?
also this is an exaple output of the renderer just a 3D cube with a texture captured using an orthographic camera
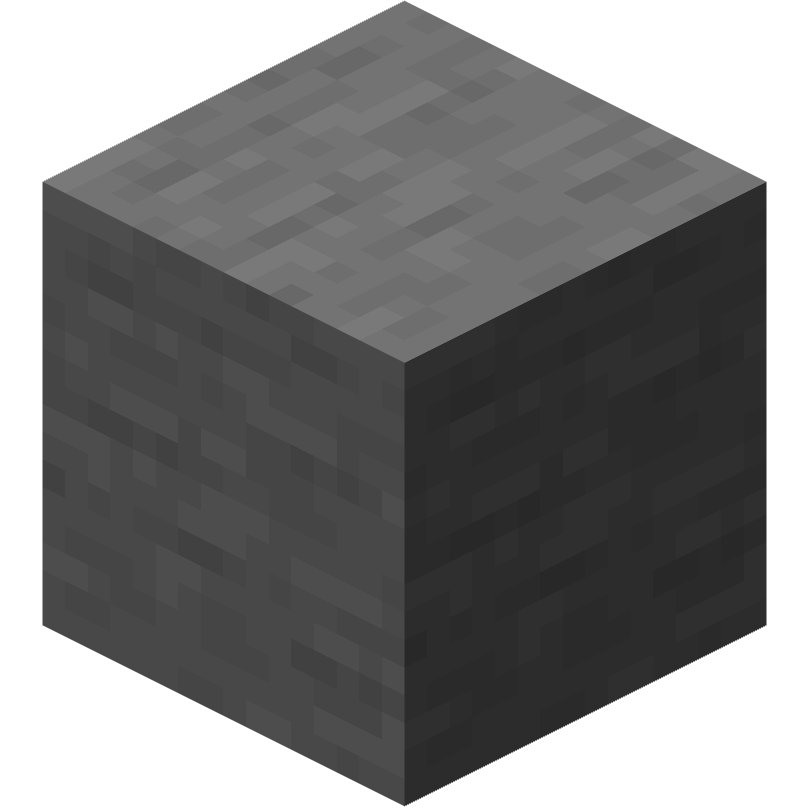
the problem is that its still 3D and it has directional light
@zamfofex any idea if there is a package that can let me render things in the deno environment instead of using html? or something that can create images with the possibility of loading textures with corner positions (idk what its officialy called) to be able to make it look 3D
or mabye be able to export the threejs render in a different way so i can access it
ohh thats cool. already figured out another way tho. mabye a bit slower but idc abb speed. i made the renderer set the textContent to the dataUrl and use puppeteer to load the page, wait for the data to be put in the body and turn the data into an image.
got another problem now is that the image wont show.
this is the code where it puts the items on the crafting grid:
getTexture function:
and here it loads the image:
the image is the result and the
recipe.json
file is the inputthe width of the stone image is also logging just fine so it clearly has the image. its just not putting it in
sorry nvm its working. had to change the image size to a standard of 16 (the width of the crafting grid)
but thx for the help. still used part of your code to get the image from the data url