How to feed a ReadableStream / async Generator into a Response object?
`` Bun.serve({
async fetch(req) {
return new Response(
async function* stream() {
// ~~~~~~~^?
yield "Hello, ";
yield Buffer.from("world!");
},
);
},
});
it is possible to feed an async generator directly into the fetch Response with Bun. With deno this does not work. I also tried to turn it into a ReadableStream with ReadableStream.from(), but no success so far. Is this the correct way to produce a ReadableStream from an async generator, or do I have to do this differently? thanks!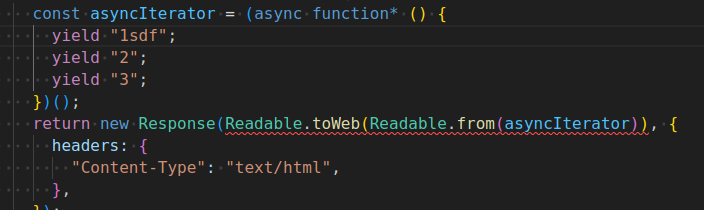
5 Replies
another try using the node:stream Readable. works in bun does not work in deno
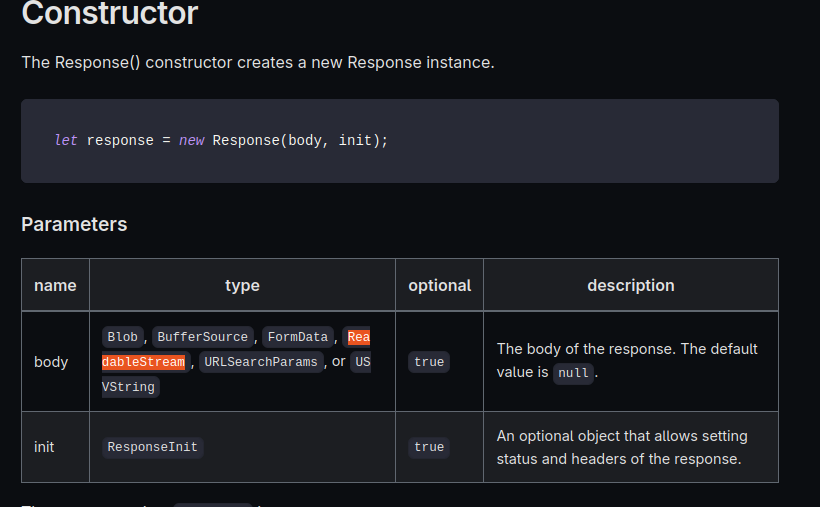
If you want to be able to mix and match strings and buffers, you need a preprocessor function like this:
Otherwise, you can get away with only buffers or only strings more easily:
thanks a lot for your reply! I didn't know yielding buffers or strings mattered. this is great info!
is there something special I need to do to flush the buffers after every yield? I tried this:
it waited 5 seconds and then sent the whole Hello world. I thought it would send Hello, then wait for 5 seconds and then send the world! 😀
(while sending the Hello instantly) EDIT: with { headers: { "content-type": "text/html; charset=utf-8" } }
it works