How to restrict global scope in dynamically created function?
I want to dynamically create a function using Function() constructor. How can I restrict access to global scope?
For example, this code:
should return something like
Uncaught ReferenceError: Deno is not defined
instead of providing full access to Deno's properties and methods.
Is it even possible?9 Replies
This is not possible
yup like luca said, it is not possible
Got it. Would be nice to see it in the roadmap 😉
A simple scenario/use-case - I want to allow my users to evaluate their ts/js script snippets in context of my object(s).
In nodejs there is another option to run some code in restricted sandbox - use vm (https://nodejs.org/api/vm.html).
In Deno I get:
Are there any plans to implement/support this?
Quoting node's documentation page about the
vm
modules https://nodejs.org/api/vm.html
The node:vm module is not a security mechanism. Do not use it to run untrusted code.
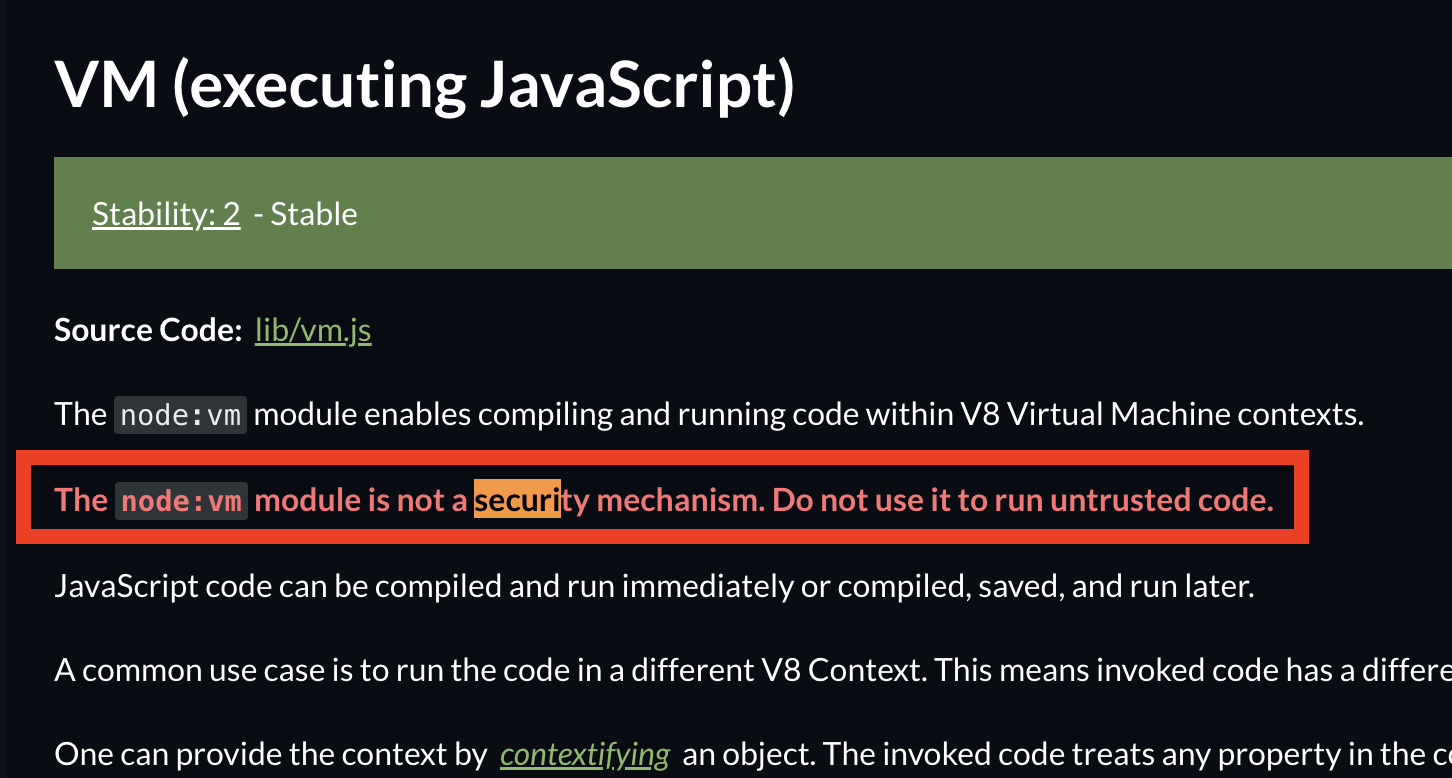
FYI: Wasn't on our radar so far that there is a bug with the second parameter. I've filed an issue https://github.com/denoland/deno/issues/22395
GitHub
Bug: Second argument to
runInNewContext
not supported · Issue #22...Input code: import * as vm from "node:vm"; vm.runInNewContext('a',{a:123}) Node: $ node Welcome to Node.js v20.11.0. Type ".help" for more information. > vm.runInNewC...
This is technically possible
but I would strongly, strongly recommend against it
If you use the deprecated
with
statement you can replace globalThis
with a proxy which allows you to define what code inside the with
statement has access toThank you. Sounds like dirty workaround.
@lino-levan
Here is a dirty trick:
This undefines all members of
globalThis
and allows access only to this
poor-man's expression evaluator 🤣interesting
even cooler:
This returns: