Uncaught (in promise) TypeError: cannot read headers: request closed
What is this? How to fix it?
18 Replies
You might want to provide some code for people to be able to help you.
The error itself is not quite useful, as it is very explicit. Request is closed, so you cannot read headers.
You are probably sending a Response, then trying to read headers from the Request.
ahm, ok, sorry.
The fact that it says "Uncaught (in promise)" hints that you might not be
await
ing a promiseas the file is very small, here is it:
which line throws the error ?
when the error occurs, both files (kv-shm and kv-wal) are deleted.
i don't know the exact line
it occurs while editing data on the client (react)
seems it has something to do with KV !??!?!
or
deno run --watch
... as script ?
i am guessing.So it fails when you do a POST request to one of the endpoint ?
Error should have a stacktrace so you can deduct where the error come from, do not hesitate to post it here
i will, just waiting for it to occur again
Oh, is it not happening every time ? Makes it quite hard 😭
yes it is.
it is rarely happening.
i think it might be a bug from early KV
as i said. both database files are missing after the error
this is the full log
maybe the problem is at the side of the react-client which breaks the connection ???
Looks like an issue with oak, where the connection dies unexpectedly
ok
Does the react client crashes or something ? Because requests ending unexpectedly when working locally seems pretty hard to do
yes.
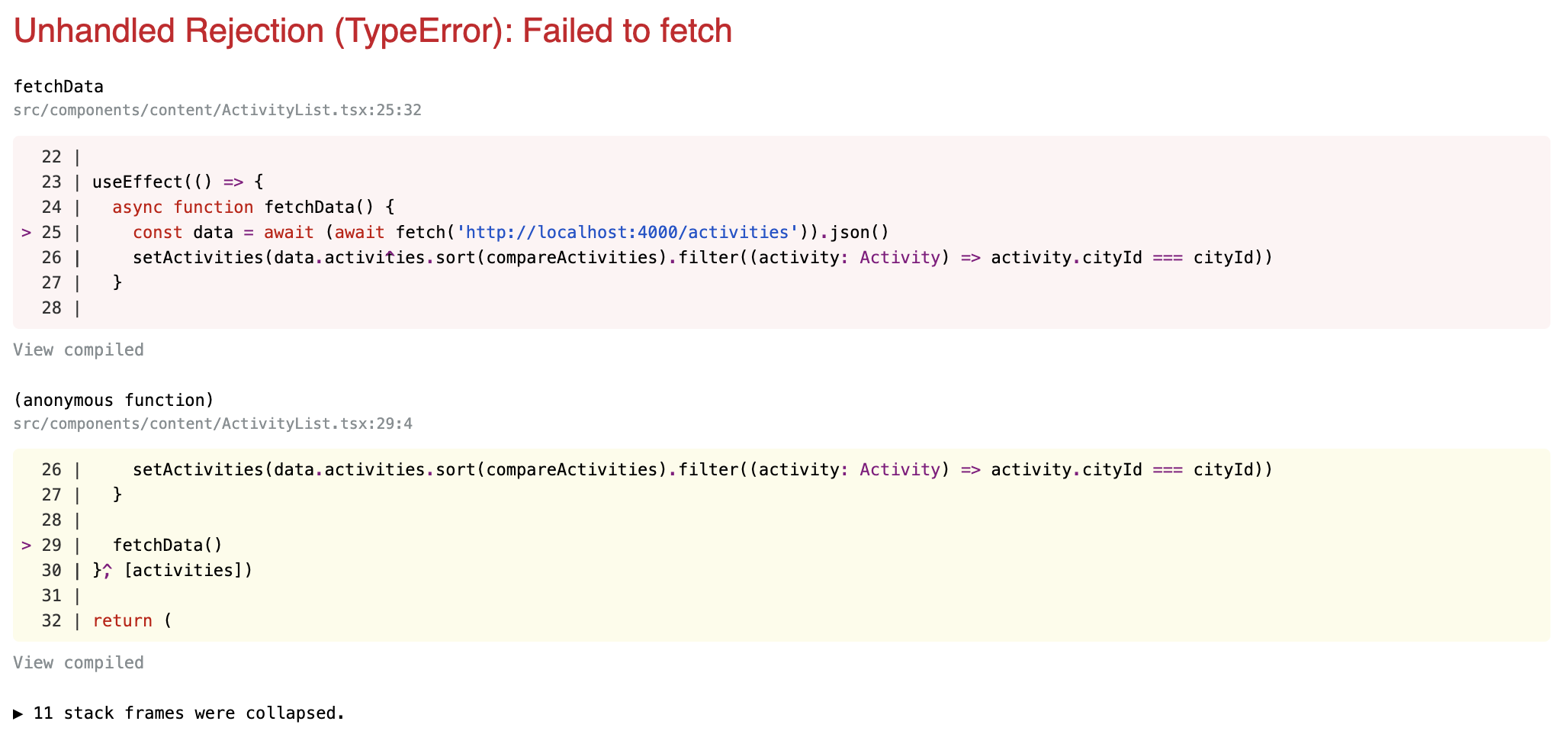
I am working with parcel as a bundler.
i am switching to vite.
let's see if that works
there seems to be a solution
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
no, I did not find a solution.