Sending proper Content-Type depending on file sent
Hello. Im new to Deno and TypeScript and i wanted to convert one of my older projects (NodeJs and JavaScript). Now, my plan is to send the requested file, which actually does work, but the response doesnt send the proper content-type. What do i have to do in order to get this working properly?
3 Replies
You'd need to place the content-type header yourself.
Consider using the file server or serve from std: Those are more "batteries included" servers.
Im now using file-server (serveDir), as you told me to, but whenever im requesting a file that doesnt exist in my fsRoot directory, im getting those weird looking messages. How can i prevent this?
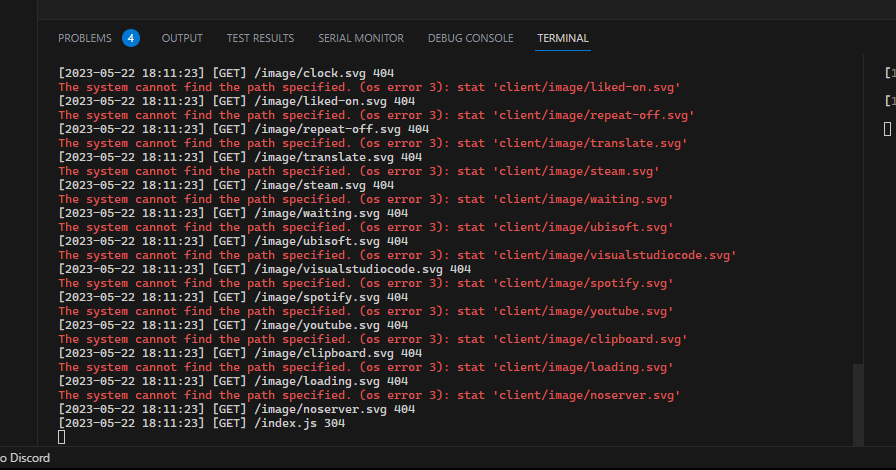
Found it out already, had to add
quiet: true
to the serverDir