Preventing `Deno.stdin` reads from blocking
In a module for reading
Deno.stdin
input, there is a loop that reads and parses the returned bytes.
My issue is that, in case of an uncaught error/rejection, the process hangs until stdin
has something to return, and once it does, only then does it exit the process and log the error information.
Is there a way to make this read()
non-blocking in that sense?
If the user does not input anything, but an error occurs, the process should exit immediately and not have to wait for a keypress.
The code below can be run from a file for demonstration.
8 Replies
(actually, there is an issue about this at https://github.com/denoland/deno/issues/14333, but it hasn't gotten any attention for about 8 months)
Which version of Deno do you use?
latest
its really infuriating because it prevents me from restarting the app in case something breaks
That is really annoying. Hop some of the core team members read this
This is likely related to / same issue as https://github.com/denoland/deno/issues/18131
GitHub
FFI: Errors cannot be thrown after a
nonblocking
function call · ...call_some_nonblocking_function(); console.debug("Reaches here."); throw new Error(); // not thrown
heh
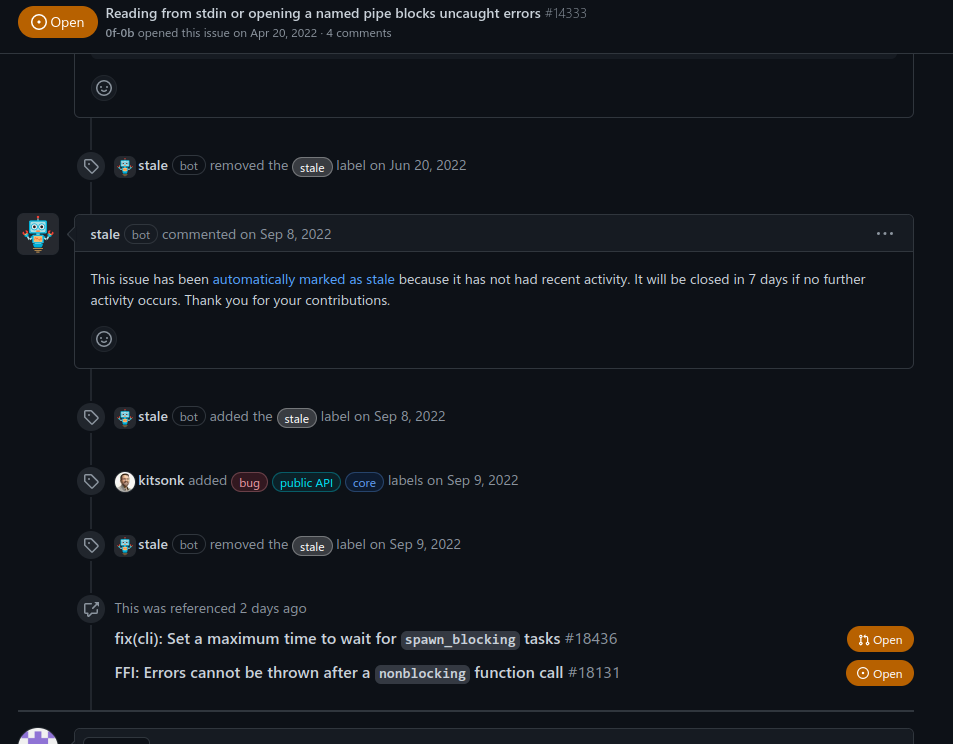
good to know it is in fact a bug
(and that it gained some attention, which i appreciate a lot)
Stalebot used to be a bit of a pain 🙂 Hence why it was removed.
Whether or not this is a bug or a feature could perhaps be debated. I would personally opt on the side of "it's a bug" but I'm sure someone could argue the opposite as well.
I'll try my best to get some attention to this but don't expect too much. It's a painful edge case with only annoying answers on offer (I believe).