How to forward a reference to a component
I created this sample to exemplify what I don't understand. Using the Fresh framework, I created the following Input component:
Inside of an island, I know I can add events listeners to the
<input />
element inside the Input component thanks to the spreading of the rest of the props ({...props}
). But when I try to add a reference to it, like so:
The reference is not forwarded to the <input />
element, instead it targets what I suppose is a component object of some sort. Definitely, the reference doesn't target the HTMLInputElement
as it should. I believe I'm forwarding the reference in an incorrect way. The attached image is what console.log(refInput.current)
logs.
Thanks in advance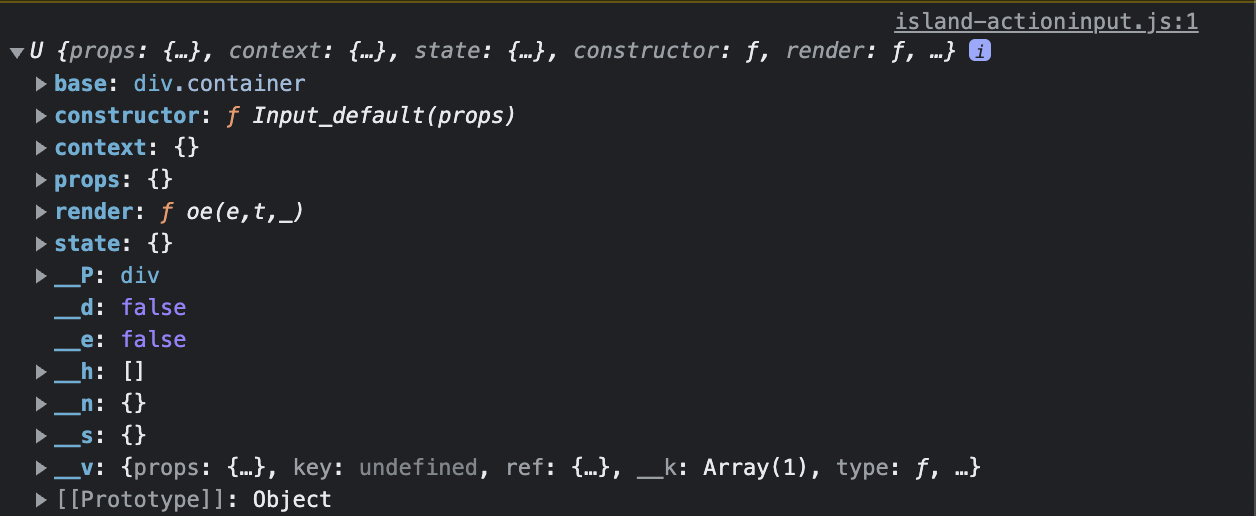
1 Reply
This shouldn't even get you div, I guess preact is a bit less strict...
Ok, aside of that... declare ref in props as any other name, f.e. inputRef, then in input element do
ref={inputRef}
In react there is "forwardRef" to make it work like you want here... maybe search "forwardRef preact" before using the first method
@.poncho. ^