axios gives me a CORS error, fetch doesn't. Why is that?
NODE
Access to fetch at 'https://example-29544e.webflow.io/' from origin 'http://127.0.0.1:5173' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled. fetcher.js?v=36de8185:44 GET https://example-29544e.webflow.io/ net::ERR_FAILED 200DENO This seems to fetch the data just fine. Interestingly, using the above approach in Node produces another CORS error. I'm confused
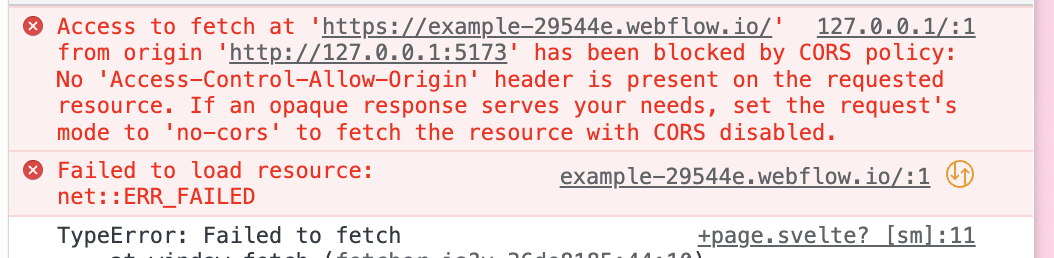
45 Replies
Deno's fetch ignores cors. The deno devs have figured that it's kinda useless to have a fetch if it cannot be used on all http links. Even if that might break cors policy
Is there any way to ignore cors in node fetch?
not that I know of
Then how do people usually fetch the data in those sort of scenarios?
idk. I don't use node enough to know this. There is probably something that does ignore cors but idk which packages that would be
Maybe you can manually set the Origin header to a value the server will accept, IDK if fetch lets you do that.
I will try the sveltekit deno adapter and see if I can get it to work there
Quick question, I just created a demo project with
deno init
added index.html
and added the main.ts
to the html head via <script src="main.ts"></script>
but I can't seem to get it to work. I keep getting Refused to execute script from 'http://127.0.0.1:5500/main.ts' because its MIME type ('video/mp2t') is not executable.
in console.
What am I doing wrong?
the dev server was started using the vsc extension live serverwell you cannot run ts in a browser / html file so the extension has to be
.js
rather than .ts
and it errors because it thinks the main.ts
file is a video and not a javascript fileIt keeps telling me that document is not defined
error: Uncaught (in promise) ReferenceError: document is not defined const el = (document.querySelector('body').innerHTML = bodyContent)and in console
Uncaught SyntaxError: Cannot use import statement outside a module (at main.js:1:1)
Put
type="module"
on your script tag.Thanks, that has gotten of the import error, but document is still undefined
This is in the browser yes?
no, when I run deno run --allow-write --allow-net main.js
Document isn’t defined in deno.
It gives you type information but there is no document in deno. Unlike a html page which is a document.
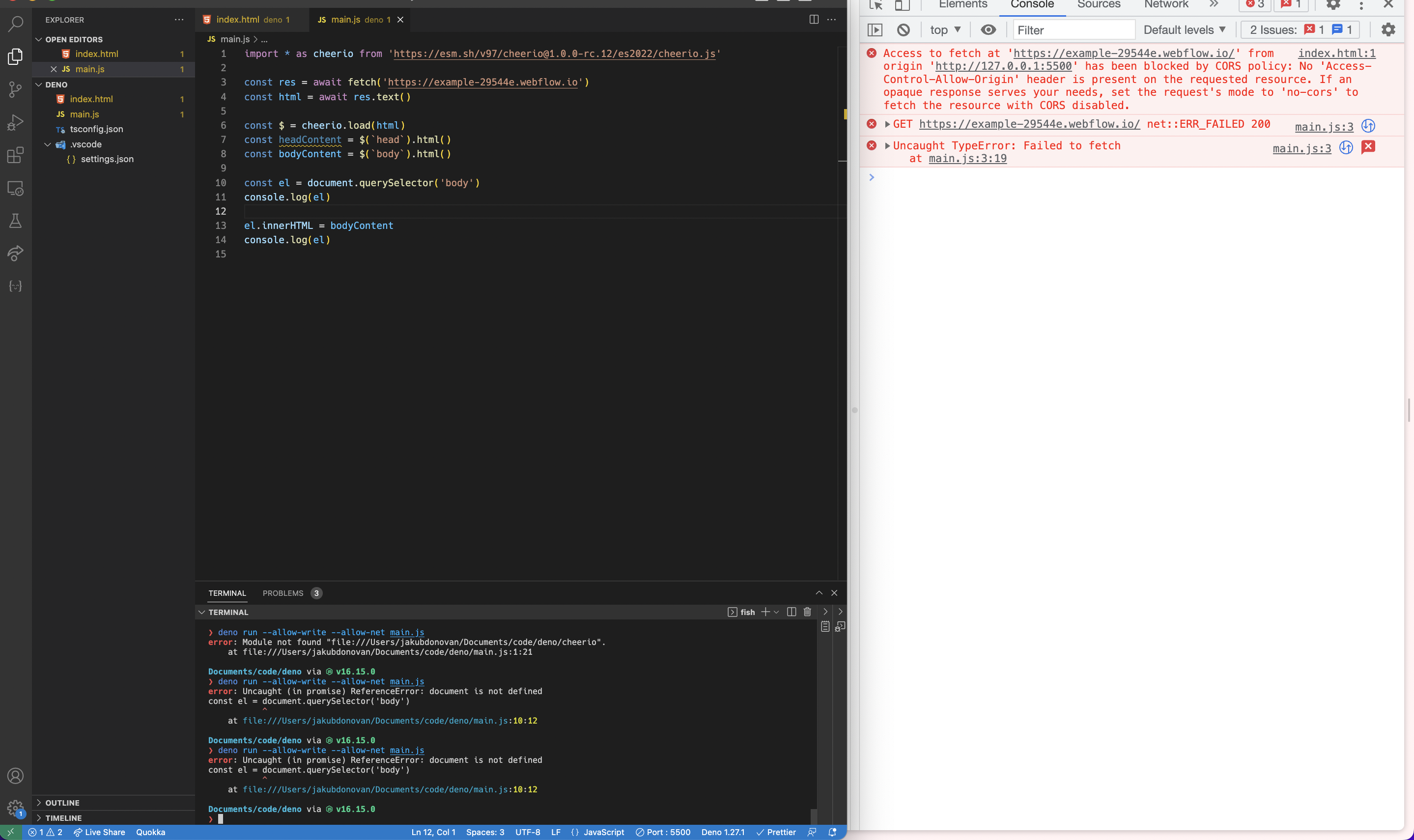
So how does one interact with the dom?
There is no dom in deno.
So deno is not meant to be used as a node replacement for web development?
The webpage has a dom. The deno instance doesn’t.
right, but I can't even access the dom like i would in js
document.querySelector and so on
If you want to access the webpage you load the index.html and it calls the main.js and runs that in the browser.
That's what I did
^
but it's not even logging the element
Because you have other errors which broke the rest from running.
I thought that Deno ignores cors
Deno does. Your web browser doesn’t.
I'm able to log the response to my terminal, but browser breaks.
You loading the index.html is completely seperate from deno.
These are two seperate instances of JavaScript running.
How do I get the browser to shut up and just do what i need it to
deno get the data and then put it into the html content of the element
You’ll need to make deno into a web server to serve the html to the client (your browser) instead of opening the index.html file manually. That way you can then do fetches to deno from the client to get whatever you want.
Is there a tutorial for this?
you mean using serve?
I haven’t used it myself but yes
lol what the hell
the page is just serving the text in the response and ignores my index.html
You didn’t send your index.html. You sent "Hello, World!"
the doc doesn't say how to
Looking at the docs you need to pass new Response a BodyInit which accepts a Blob, BufferSource, FormData, URLSearchParams, ReadableStream<Uint8Array>, or a string.
So you’ll need to open the index.html file in deno and send it in the response.
You can open a file with Deno.open and turn it into a ReadableStream<Uint8Array> with .readable.
This is terrible and not at all beginner friendly.
I'm just gonna use fresh
¯\_(ツ)_/¯
must be doing it wrong?
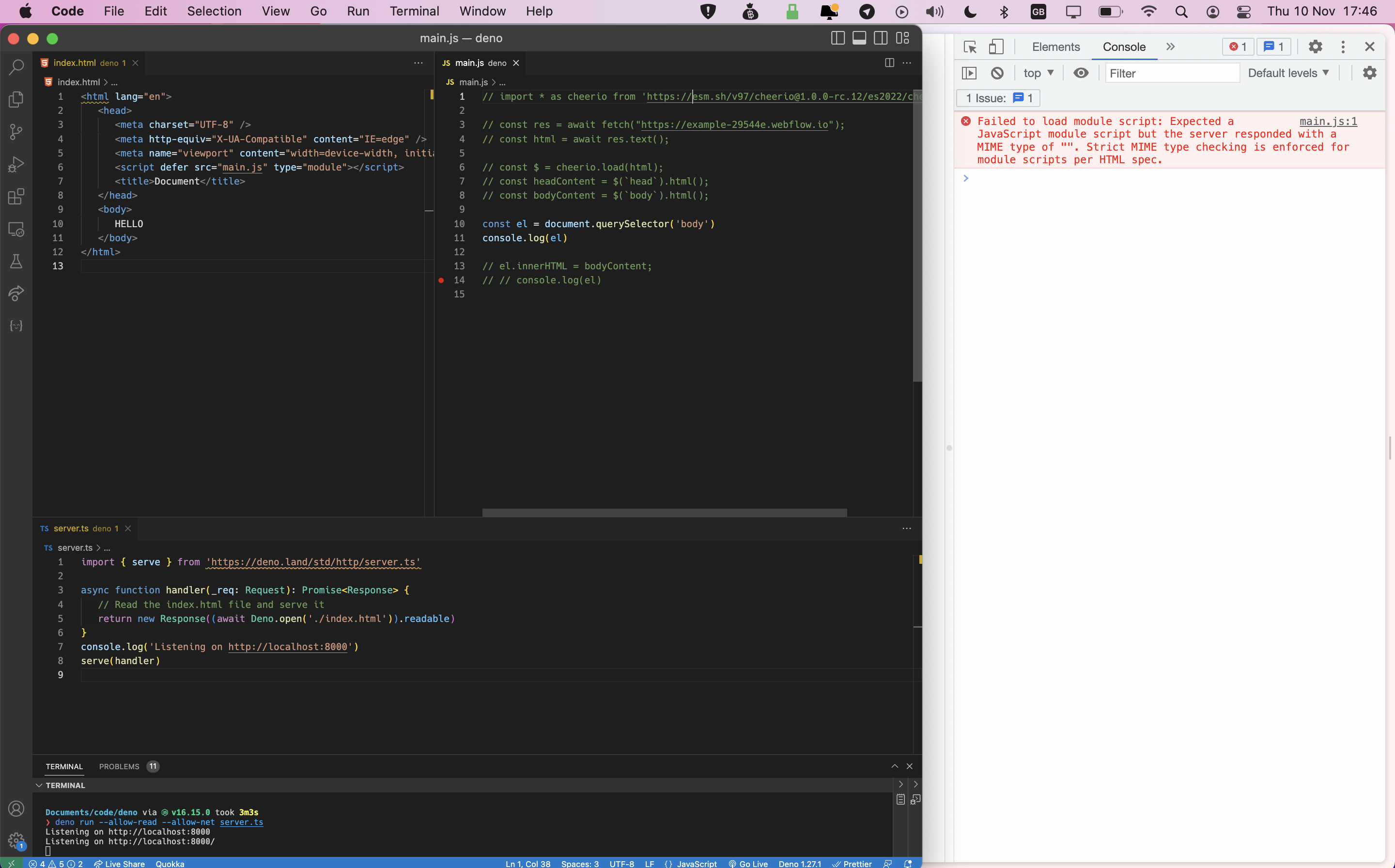
Deno is serving index.html regardless of what url you request so when the page requests main.js it gets index.html again.
Of the req in the handler, you’ll need to look at the url and give the correct response based off that.
@dr_light @ndh3193 even so, none of the code in my
main.js
executes and the body gets logged to console even though I am not console.logging it anywhere. I'm super confused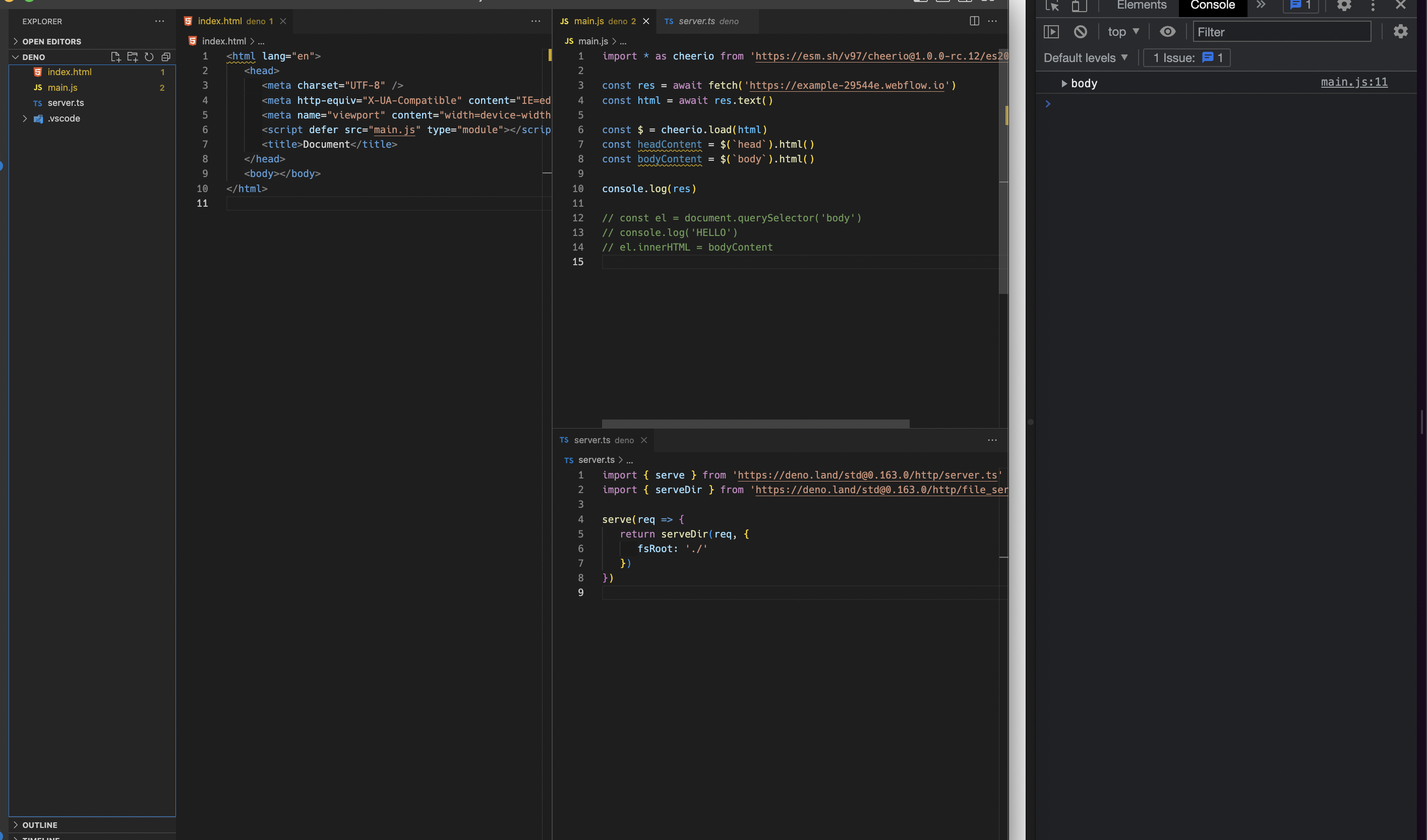
Did you save main.js? Try doing a hard refresh.
Cmd + shift + R in the browser.
restarted the localhost multiple times as well
That seems to have worked